Interact with Salesforce using PHP
Interact with Salesforce using OAuth 2.0 Authorization Code Grant Type.
I know the fact that to have a thorough understanding of OAuth 2.0 and IAM is a difficult thing, and still thought of myself lack some on-hands experiences on this area.
When I was preparing the Identity management exam, I came across this great article OAuth-Authorization-Code-Grant-Type which theoretically explained the Authorization Code Grant Type in detail. I read through these materials and found I need to do a real-world practice to reinforce my understanding. And finally I found this one: interact-with-the-forcecom-rest-api-from-php Although it is an old post,the specification behind the scenes still hasn't changed today in my opnion.
I think the data flow of this demo is like this(can be found in this blogpost: Digging_Deeper_into_OAuth_2.0_on_Force.com ), which is the Web Server Flow.
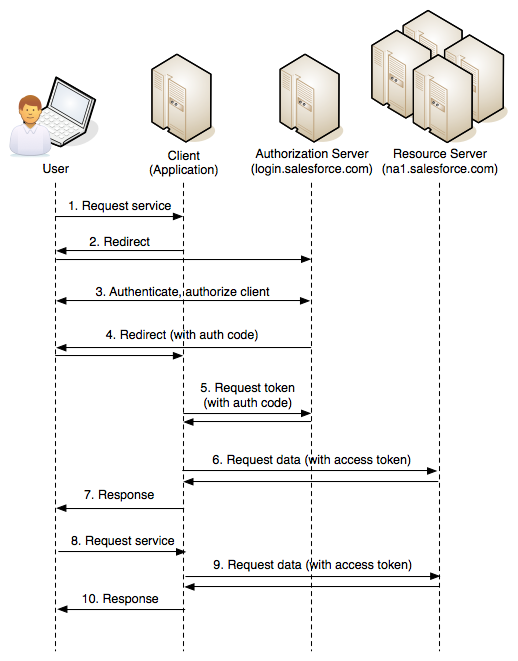
How is the authenticating with OAuth implemented?
There is one prerequisite need to do: enabling SSL on a Apache webserver. The commands setting the SSL using the public key: X509 are:
- sudo openssl req -new -key /etc/apache2/server.key -subj "/C=/ST=/L=/O=/CN=/emailAddress=/" -out /etc/apache2/server.csr
- sudo openssl req -new -key /etc/apache2/ssl/localhost.key.rsa -subj "/C=/ST=/L=/O=/CN=localhost/" -out /etc/apache2/ssl/localhost.csr -config /etc/apache2/ssl/localhost.conf
- sudo openssl x509 -req -days 365 -in /etc/apache2/server.csr -signkey /etc/apache2/server.key -out /etc/apache2/server.crt
- sudo openssl x509 -req -extensions v3_req -days 365 -in /etc/apache2/ssl/localhost.csr -signkey /etc/apache2/ssl/localhost.key.rsa -out /etc/apache2/ssl/localhost.crt -extfile /etc/apache2/ssl/localhost.conf
You can find these steps on the web using Google.
Here is the ultimate result on my local machine.

The core part of the code: oauth_callback.php
require_once 'config.php';
session_start();
$token_url = LOGIN_URI . "/services/oauth2/token";
$code = $_GET['code'];
if (!isset($code) || $code == "") {
die("Error - code parameter missing from request!");
}
$params = "code=" . $code
. "&grant_type=authorization_code"
. "&client_id=" . CLIENT_ID
. "&client_secret=" . CLIENT_SECRET
. "&redirect_uri=" . urlencode(REDIRECT_URI);
$curl = curl_init($token_url);
curl_setopt($curl, CURLOPT_HEADER, false);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, $params);
$json_response = curl_exec($curl);
$status = curl_getinfo($curl, CURLINFO_HTTP_CODE);
if ( $status != 200 ) {
die("Error: call to token URL $token_url failed with status $status, response $json_response, curl_error " . curl_error($curl) . ", curl_errno " . curl_errno($curl));
}
curl_close($curl);
$response = json_decode($json_response, true);
$access_token = $response['access_token'];
$instance_url = $response['instance_url'];
if (!isset($access_token) || $access_token == "") {
die("Error - access token missing from response!");
}
if (!isset($instance_url) || $instance_url == "") {
die("Error - instance URL missing from response!");
}
$_SESSION['access_token'] = $access_token;
$_SESSION['instance_url'] = $instance_url;
header( 'Location: demo_rest.php' ) ;
The last but not the least, there are plenty of good videos on the youtube:
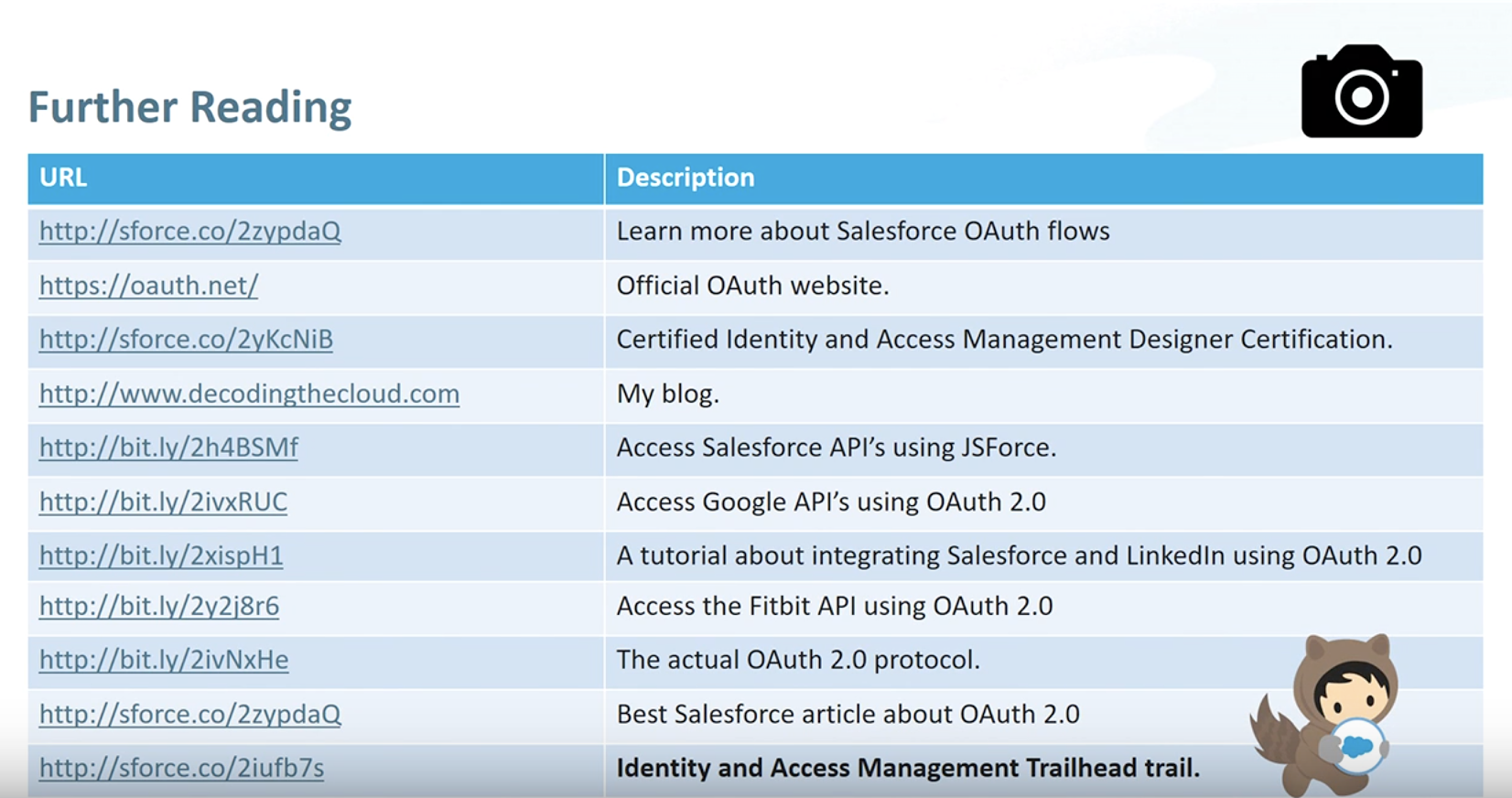